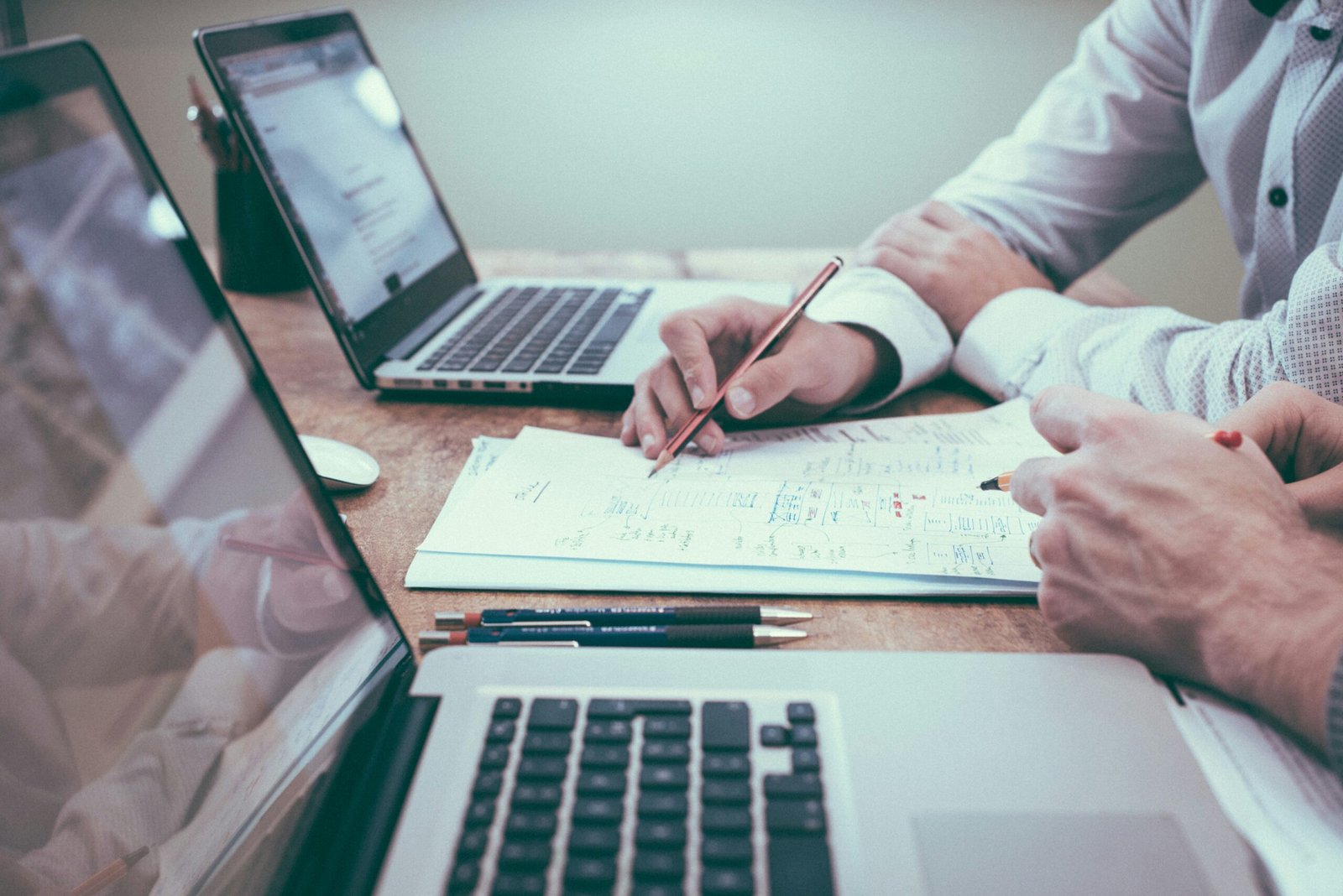
Helper and Service Classes in PHP
Helper and Service classes are widely used in PHP applications to improve code organization, maintainability, and reusability. Both serve different purposes but sometimes overlap in functionality.
1. Helper Class
A Helper class contains static utility functions that perform generic tasks. These functions usually do not require object instantiation and are stateless.
Characteristics of Helper Classes
✅ Collection of utility methods.
✅ Typically static methods (no need for object instantiation).
✅ Stateless (does not maintain any data).
✅ Used across the application wherever needed.
Example of a Helper Class
class StringHelper {
public static function toCamelCase(string $string): string {
$string = str_replace(' ', '', ucwords(str_replace('_', ' ', $string)));
return lcfirst($string);
}
public static function toSnakeCase(string $string): string {
return strtolower(preg_replace('/[A-Z]/', '_$0', lcfirst($string)));
}
}
// Usage:
echo StringHelper::toCamelCase('hello_world'); // Output: helloWorld
echo StringHelper::toSnakeCase('helloWorld'); // Output: hello_world
Here, StringHelper
provides utility methods to convert strings between different cases.
2. Service Class
A Service class encapsulates business logic and typically operates on data. Unlike Helper classes, Service classes often require object instantiation and may have dependencies.
Characteristics of Service Classes
✅ Encapsulates business logic.
✅ Operates on application data.
✅ Can have dependencies (e.g., Database, API, other services).
✅ Not static – instantiated via dependency injection.
✅ Typically used within frameworks (e.g., Laravel, Symfony).
Example of a Service Class
class UserService {
protected $db;
public function __construct(Database $db) {
$this->db = $db;
}
public function getUserById(int $id): ?array {
return $this->db->query("SELECT * FROM users WHERE id = ?", [$id])->fetch();
}
public function createUser(string $name, string $email): bool {
return $this->db->query("INSERT INTO users (name, email) VALUES (?, ?)", [$name, $email]);
}
}
Usage:
$db = new Database();
$userService = new UserService($db);
$user = $userService->getUserById(1);
print_r($user);
UserService
requires a Database instance (Dependency Injection).- It performs business logic operations like fetching and creating users.
Key Differences
Feature | Helper Class | Service Class |
---|---|---|
Purpose | Utility functions | Business logic |
Stateful? | No (stateless) | Yes (stateful, operates on data) |
Static? | Usually static methods | Typically instantiated |
Dependencies? | No | Yes (e.g., database, API, other services) |
Usage | Utility tasks (e.g., string manipulation, date formatting) | Core business operations (e.g., user authentication, order processing) |
When to Use Each?
✅ Use a Helper Class when:
- You need reusable utility functions (e.g., string, date, array helpers).
- No need to maintain state.
- Functions are pure (always return the same output for the same input).
✅ Use a Service Class when:
- You need to encapsulate business logic.
- You have dependencies (e.g., database, APIs, other services).
- The logic operates on data.
Conclusion
- Helper classes provide utility functions (stateless, often static).
- Service classes contain business logic (stateful, instantiated, operates on data).
- Both improve code structure and maintainability when used correctly.
RELATED POSTS
View all